Game Development with C++
- Live Online Weekend / Evening Classes
- 5 to 6 weeks, 10 - 12 hours per Week
- Game Development
- Intermediate
- Medium
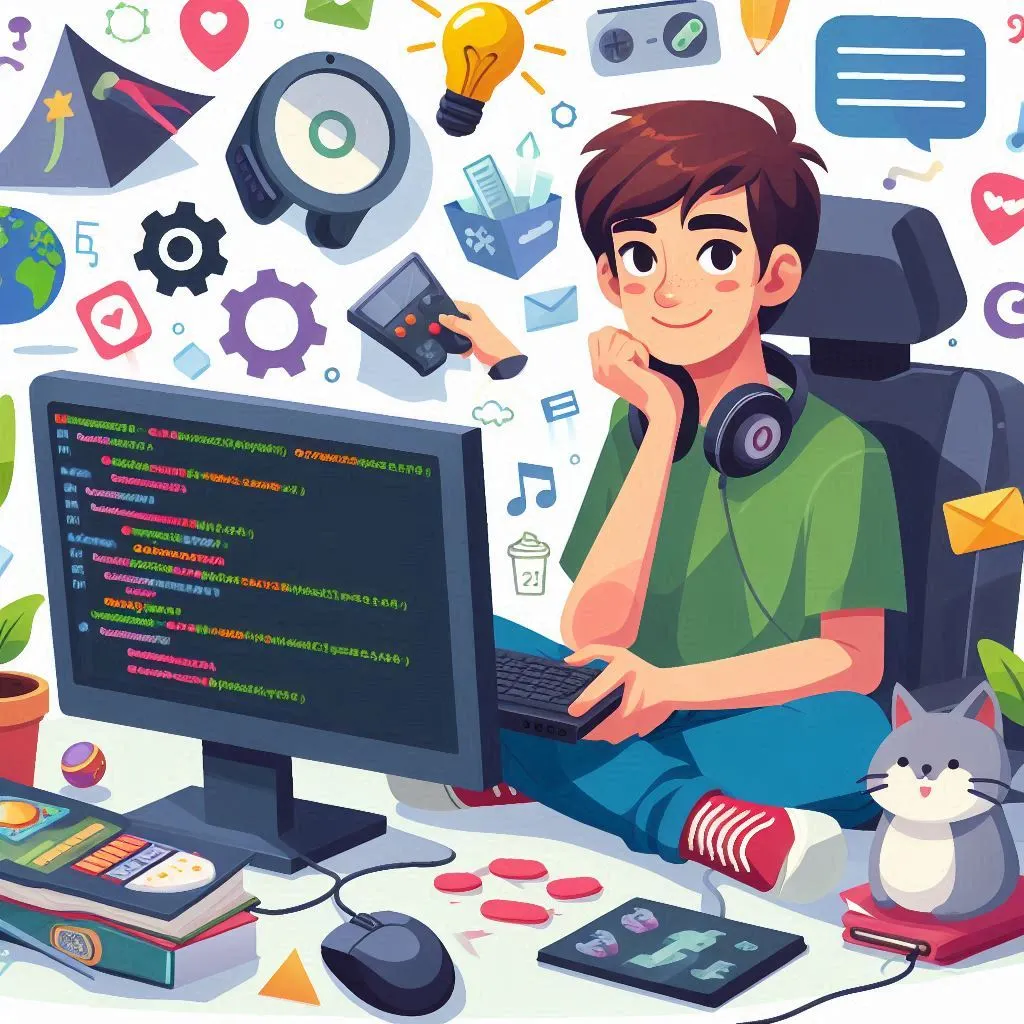
Game Loop: Understand how to structure a game loop with input handling, updating game logic, and rendering.
Memory Management: C++ memory management (manual and automatic) will be essential for performance.
2D Graphics and Physics: Master the basics of rendering, collision detection, and simple game physics.
Syllabus
● Variables, data types, and operators
● Control flow, Functions, arrays, and basic I/O
● Pointers and references
● Introduction to classes and objects
● Classes, objects, and constructors/destructors
●Encapsulation, inheritance, and polymorphism
●Overloading operators and functions
●Introduction to the Standard Template Library (STL)
● Memory management (new, delete, smart pointers)
● C++11/14/17 features (e.g., lambda functions, range-based loops)
● Exception handling (try-catch)
● File I/O and basic serialization
● Install and set up a C++ IDE (e.g., Visual Studio, CLion)
● Install and set up a game engine (like Unreal Engine or SFML)
● Introduction to debugging techniques in C++
● Writing and compiling your first C++ console application
● Install SFML and create a window
● Handle events (keyboard, mouse)
● Rendering basic shapes (rectangles, circles, etc.)
● Load and display textures (images)
● Implementing the game loop (render, update, input)
● Handling frame rate and time management
● Simple physics (movement, gravity, collisions)
● Handling player input (keyboard, mouse)
● Playing sound effects (SFML audio module)
● Implementing simple UI elements (score, health)
● Design and start a simple 2D game (e.g., a basic "Pong" or "Breakout")
● Implementing the game loop, collision detection, and player controls
● Animation (spritesheets)
● Handling different game states (menu, gameplay, game over)
● Adding background music and sound effects
● Managing game assets efficiently
● Organizing code for scalability (GameManager, Player, Enemy classes)
● Implementing Game Design Patterns (e.g., Singleton for managing game state)
● Using multiple classes for better code structure
● More advanced physics (velocity, acceleration)
● Basic AI for enemies (patrolling, chasing, simple state machines)
● Implementing pathfinding (e.g., A* algorithm for enemy movement)
● Finish building a simple 2D game (e.g., a "Space Shooter" or "Platformer")
● Implementing level design, scoring system, and game-over conditions
● Polish and debug the game
Week 1
Week 2
Week 3
Week 4
Week 5
Week 6