Java and Database Programming Projects
- Live Online Weekend / Evening Classes
- 5 to 6 weeks, 10 - 12 hours per Week
- Data Base
- Intermediate
- Medium
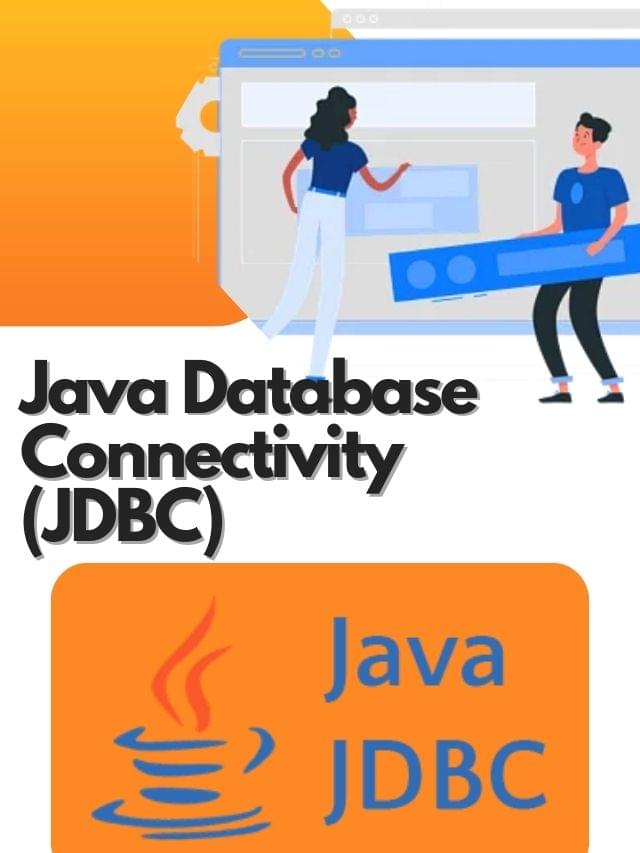
This curriculum is designed to help you learn in a structured way while focusing on practical application development.
1. A solid understanding of JDBC and database operations in Java.
2. Experience with both relational (MySQL/PostgreSQL) and NoSQL (MongoDB) databases.
3. Hands-on experience in building a complete Java database application (console, GUI, or web-based).
4. Knowledge of advanced Java database techniques such as transaction management and connection pooling.
IDE: IntelliJ IDEA, Eclipse, or NetBeans.
Database: MySQL or PostgreSQL for relational databases, MongoDB for NoSQL.
JDBC Driver: MySQL JDBC Driver (for MySQL), or PostgreSQL JDBC Driver.
Libraries: Hibernate, HikariCP (for connection pooling).
Week 1
Week 2
Week 3
Week 4
Week 5
Week 6